Display a list of items commonly will refer to a widget, named ListView. In this post, I will show you how to make a simple ListView in Flutter by creating a display-only note application.
ListView in Flutter
ListView is one of the most commonly-used in mobile apps development. Basically, there are a lot of items in the same type, same way of displaying; so ListView is fundamentals.
To demonstrate how ListView works, we will try to create a simple ListView in Flutter that displays a list of text.
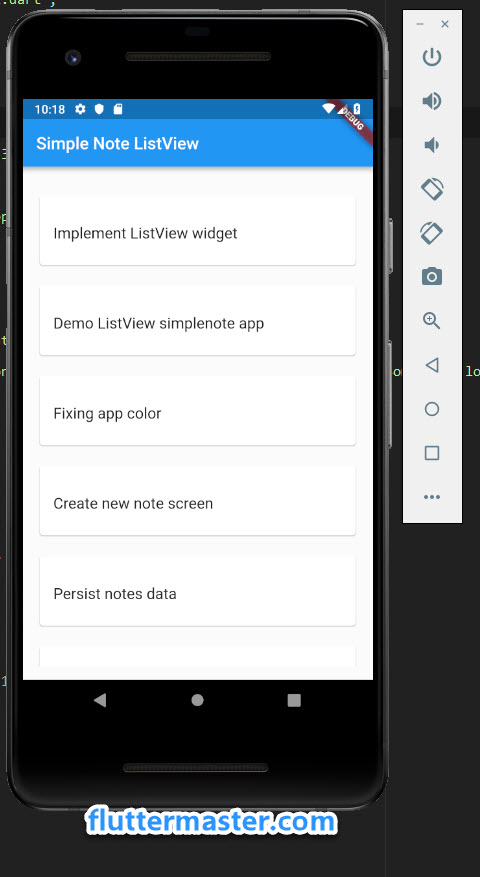
First, we need to setup a basic Flutter app to start with.
import 'package:flutter/material.dart';
void main() {
List<String> notes = [
"fluttermaster.com",
"Update Android Studio to 3.3",
"Implement ListView widget",
"Demo ListView simplenote app",
"Fixing app color",
"Create new note screen",
"Persist notes data",
"Add screen transition animation",
"Something long Something long Something long Something long Something long Something long",
];
runApp(MaterialApp(
home: Scaffold(
appBar: AppBar(
title: Text("Simple Note ListView"),
),
body: Container(
color: Colors.white10,
padding: EdgeInsets.all(16.0),
child: HomePage(notes)
),
),
));
}
class HomePage extends StatelessWidget {
final List<String> notes;
HomePage(this.notes);
@override
Widget build(BuildContext context) {
//TODO build ListView here
}
}
The structure is simple, our main code of creating ListView will be put into HomePage
class.
In order to render a list of items, we need to input a list of items into the widget for rendering.
To create a ListView, we use ListView.builder() . There is only one required parameter itemBuilder
, which is a callback function that defines how to create list item widget for ListView.
In this case, each list item displaying a simple text, we will start with only Text widget.
return ListView.builder(
itemBuilder: (context, idx) {
return Text(notes[idx]);
}
};
Reload the app, we will have this.
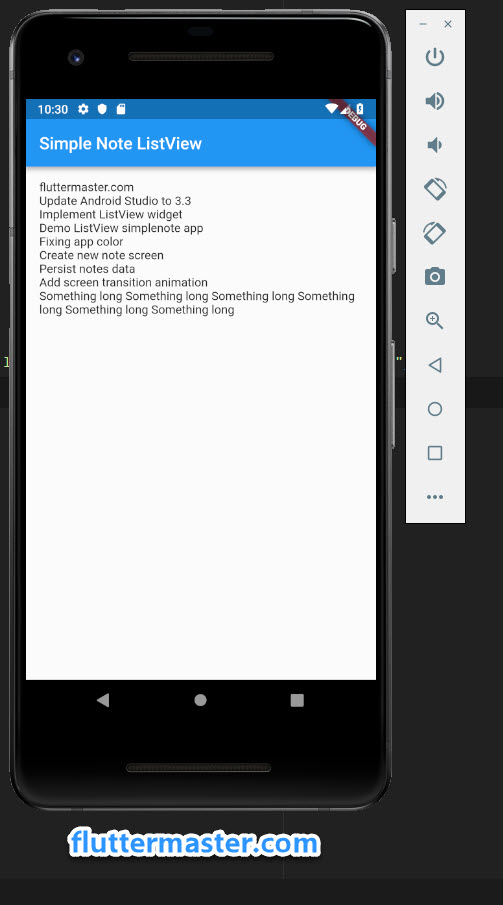
Because the list of items is too short and text style is quite small, so it fits in single screen; therefore, we cannot try the scrolling.
By default, when you use ListView.builder
to create ListView, the scroll is implemented automatically when ListView height is greater than viewport. You can try by adding more items in to notes
and increase font size for the text.
Another important parameter is itemCount
to calculate maximum range of scroll for the ListView. If you don't provide this value, the ListView widget will self-calculate the maximum extent for the scroll. The recommendation is to always use the number of list items to render for itemCount
.
At this point you should have added several UI customization for the ListView and its children already.
This is how I customize the ListView:
return ListView.builder(
itemCount: notes.length,
itemBuilder: (context, pos) {
return Padding(
padding: EdgeInsets.only(bottom: 16.0),
child: Card(
color: Colors.white,
child: Padding(
padding: EdgeInsets.symmetric(vertical: 24.0, horizontal: 16.0),
child: Text(notes[pos], style: TextStyle(
fontSize: 18.0,
height: 1.6,
),),
),
)
);
},
);
Not so cool but it is enough for a simple note app.
Summary
It is fundamental to know ListView since you will have to deal with it in almost every mobile apps, so take your time to learn the basics.
The code for the simple ListView in Flutter is posted here for your reference, https://github.com/petehouston/flutter-tips/tree/master/simple-listview