If you think it is complicated to create a horizontal scrolling widget in Flutter, you are wrong. In facts, it is easy to create horizontal ListView in Flutter.
By horizontal scrolling, I mean this.
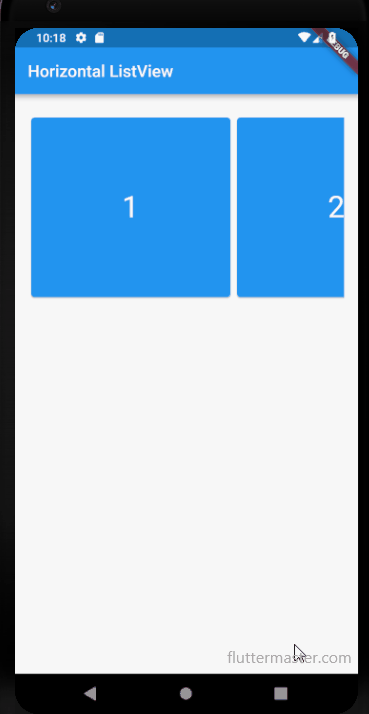
The widget is the ListView that I introduced before, "Make simple ListView in Flutter".
The only difference is in the way you config the list through scrollingDirection
property, which can be either Axis.vertical
or Axis.horizontal
. And that's it!
The rest of the ListView UI building is the same.
Quick Tip: you might need to config the width and height of horizontal ListView, because you might want users to know that there is some parts of view can be scrolled horizontally.
Try to avoid full-width when implementing horizontal ListView.
Try to avoid full-width when implementing horizontal ListView.
This is the complete code for demonstration of making horizontal listview in Flutter.
import 'package:flutter/material.dart';
void main() => runApp(MaterialApp(
home: MainScreen(),
));
class MainScreen extends StatelessWidget {
final List<int> numbers = [1, 2, 3, 5, 8, 13, 21, 34, 55];
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: Text('Horizontal ListView'),
),
body: Container(
padding: EdgeInsets.symmetric(horizontal: 16.0, vertical: 24.0),
height: MediaQuery.of(context).size.height * 0.35,
child: ListView.builder(
scrollDirection: Axis.horizontal,
itemCount: numbers.length, itemBuilder: (context, index) {
return Container(
width: MediaQuery.of(context).size.width * 0.6,
child: Card(
color: Colors.blue,
child: Container(
child: Center(child: Text(numbers[index].toString(), style: TextStyle(color: Colors.white, fontSize: 36.0),)),
),
),
);
}),
),
);
}
}