Almost every single mobile app will have a splash screen when start. Let’s create splash screen in Flutter.
Splash screen in Flutter
First, let’s recap some characteristics of splash screen.
- It is for branding and identity recognition. Splash screen helps to put branding impression to users.
- Often, app will need to pre-fetch data required for app to be able to use, ex: loading data from servers, verifying user authentication and identity…
- It might show some loading progress indicator to let users know that is loading something.
- Once splash screen completes, it transits to the next screen, often to be home screen or dashboard, then is forgotten. It means, pressing back button won’t return to splash screen.
Okay, so let’s implement it in Flutter.
This is the simple splash screen.
class SplashScreen extends StatefulWidget {
@override
State<StatefulWidget> createState() {
return SplashScreenState();
}
}
class SplashScreenState extends State<SplashScreen> {
@override
void initState() {
super.initState();
}
@override
Widget build(BuildContext context) {
return Container(
decoration: BoxDecoration(
image: DecorationImage(
image: AssetImage('assets/splash.jpg'),
fit: BoxFit.cover
) ,
),
child: Center(
child: CircularProgressIndicator(
valueColor: AlwaysStoppedAnimation<Color>(Colors.redAccent),
),
),
);
}
}
Basically, it is a full-display image widget, nothing more. Use can either make a colorful screen or using a fullscreen image for splash screen purpose.
If you prefer to use image, then it’s best to load image from assets, because it is packed with the app distribution package and is loaded very quick.
Also, applying a loading progress circle will help users knowing that app is loading will improve overall user experiences. CircularProgressIndicator is definitely a good choice, or you can customize something better.
Okay, so assuming we are trying to implement some loading actions when splash screen starts, let’s create a dummy method to countdown around 5 seconds, then it will navigate to home screen.
@override
void initState() {
super.initState();
loadData();
}
Future<Timer> loadData() async {
return new Timer(Duration(seconds: 5), onDoneLoading);
}
onDoneLoading() async {
Navigator.of(context).push(MaterialPageRoute(builder: (context) => HomeScreen()));
}
As you can see, the above code uses Timer for countdown operation, and after 5 seconds reach, it triggers the onDoneLoading()
action.
Again, to navigate to next screen, we use Navigator.push()
.
If you try to run above code, it will work fine, just one problem, “pressing back button make it return to splash screen“. We don’t want this behavior. How to fix it?
Navigator.pushReplacement()
is designed for this. It will move to new screen and remove previous screen from navigation history stack. Hence, the update for navigation part is:
onDoneLoading() async {
Navigator.of(context).pushReplacement(MaterialPageRoute(builder: (context) => HomeScreen()));
}
Finally, we have a pretty cool splash screen in Flutter apps.
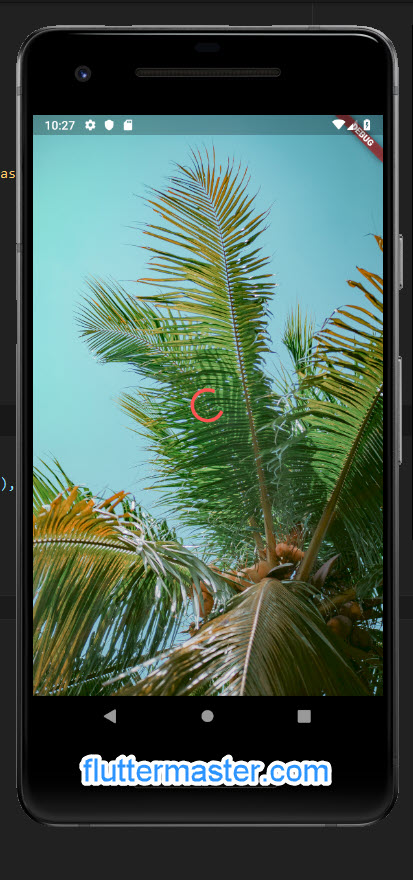
Summary
Splash screen is just another fullscreen widget you need to build, and it’s easy. Hope you like this Flutter tutorial.
For references, the code for the tutorial is put on Github, https://github.com/petehouston/flutter-tips/tree/master/create-splash-screen
Photo credits to Han Lahandoe at Unsplash.