Navigation system is one of the most important in any mobile app development framework. So in this article, I will guide you on how to navigate between screens in Flutter.
Navigate between screens in Flutter
When you import, use and build widgets from package:flutter/material.dart
package, your screens, commonly derived from Scaffold
class, is already under navigation stack management by Flutter.
Create a Flutter app
In this tutorial, we will try to move back and forth from a screen to another screen. Let's start by creating an app.
import 'package:flutter/material.dart';
void main() => runApp(App());
class App extends StatelessWidget {
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Flutter Navigation',
home: MainPage(),
);
}
}
Create a main page
The main page will contain only one button, when users click the button, it will move to another screen, called it sub-page. We start by designing the UI first.
class MainPage extends StatelessWidget {
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: Text('Main Page'),
),
body: Center(
child: Column(
mainAxisAlignment: MainAxisAlignment.center,
children: <Widget>[
Text('Click button to move to SubPage'),
RaisedButton(
textColor: Colors.white,
color: Colors.blue,
child: Text('Go to SubPage'),
onPressed: () {
// TODO
},
)
],
),
),
);
}
}
Create a sub page
Next, we need to create a sub-page, that is opened from main page. On sub-page, there has a button, when click it returns to main page.
class SubPage extends StatelessWidget {
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: Text('Sub Page'),
backgroundColor: Colors.redAccent,
),
body: Center(
child: Column(
mainAxisAlignment: MainAxisAlignment.center,
children: <Widget>[
Text('Click button to back to Main Page'),
RaisedButton(
textColor: Colors.white,
color: Colors.redAccent,
child: Text('Back to Main Page'),
onPressed: () {
// TODO
},
)
],
),
),
);
}
}
Navigate from main page to sub page
Let's talk about navigation. Navigation in Flutter is done by Navigator class.
Because the history is arranged in a stack structure, so moving to another screen means to launch a new instance of that full-screen widget.
We will use push()
for that.
Update to //TODO
part in MainPage
class above.
onPressed: () {
navigateToSubPage(context);
},
and add new method for MainPage
to handle navigation.
Future navigateToSubPage(context) async {
Navigator.push(context, MaterialPageRoute(builder: (context) => SubPage()));
}
Navigate back from sub page to main page
So, we are at sub page. We need to move to main page.
The first thing you can notice is the Back
icon button on app bar in sub page. It is auto-handled by Flutter navigation system.
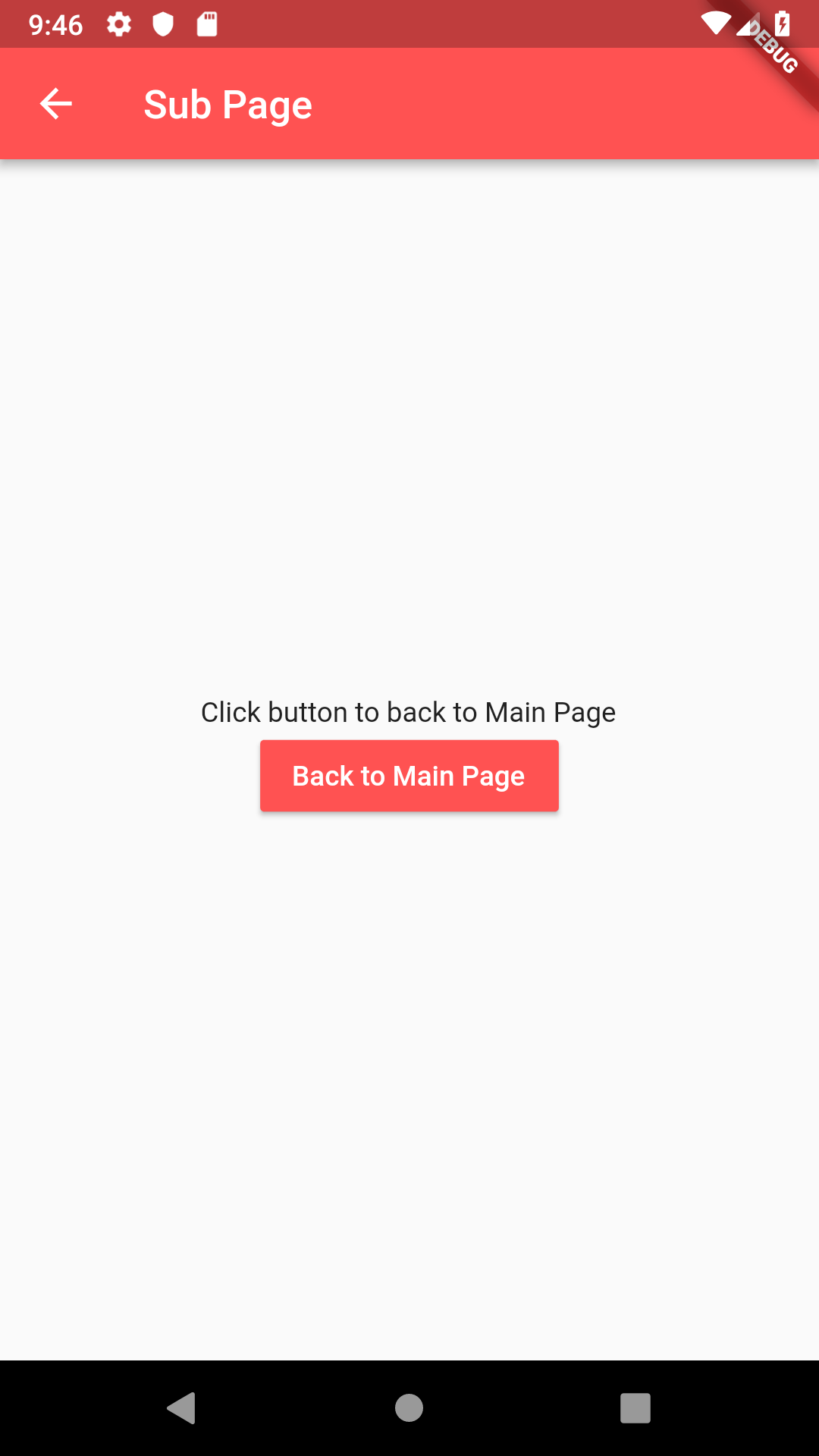
Also, you will need a method to manually control the navigation in your app, right?
What you need to do is to pop from history stack, and it will redirect us to previous screen in Flutter.
It can easily done by this call in //TODO
part of SubPage
class.
Navigator.pop(context);
then, boooooom...you're back to the first screen.
Summary
The navigation system in Flutter is super-easy to use for developers as you can see from this tutorial.
It takes only 2 lines of code to navigate between screens in Flutter; well, for this example. Everyone can do it when coding Flutter first time.
All source code demo for this tutorial is here at Github, https://github.com/petehouston/flutter-tips/tree/master/navigate-between-screens
Check it out if you need them.