Commonly, you will need to display images in assets to the application. I will show you how to do load image from assets in Flutter the easy way.
Load image from assets in Flutter
In general, to load image from assets, you will need to follow these steps:
- Create an assets directory and put in static images.
- Define assets to be used in
pubspec.yaml
- Load image from assets in code.
Okay, so let’s do it.
Step 1: Create asset directory
At the root of project, create a new directory to store images, you can name it like images
.
However, in practice, developers often need to store many types of different assets besides images, like fonts, text … so it is better to create a general asset directory then separate them out. Image is not exception.
Therefore, I recommend to create the asset directory name assets
then create a sub-directory named images
to store all required images in the app.
The directory structure will be similar to this:
flutter_project
|--assets
|--images
|-- image_01.png
|-- image_02.jpg
Step 2: Define assets
We need to specify what asset files will be used in the apps by defining them in pubspec.yaml
file.
Scroll down to the flutter:
key , you will see there is a comment part of assets:
.
Similar to this:
# assets:
# - images/a_dot_burr.jpeg
# - images/a_dot_ham.jpeg
Un-comment them and update like following:
assets:
- assets/images/image_01.png
- assets/images/image_02.jpg
Save pubspec.yaml
file. Images are ready to be used in apps.
Step 3: Load image from assets
To load image from assets, we will use Image component and use asset() method to load image.
The input parameter for asset()
is the asset name we defined in pubspec.yaml
in assets
section.
So to load images in above case, we will call like this:
Image.asset('assets/images/image_01.png')
Refresh the code, if everything is correct then image should be shown on the app.
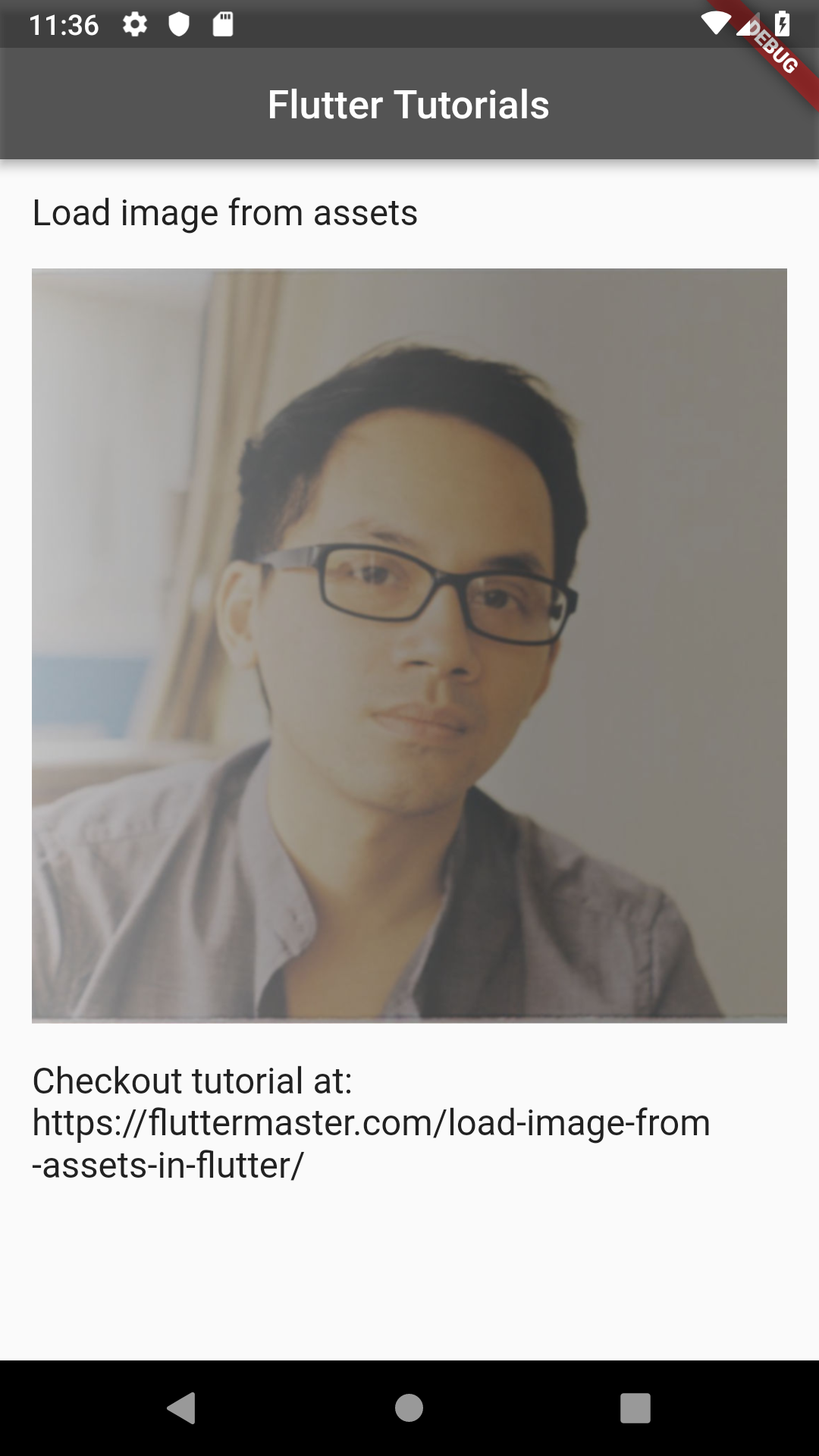
Summary
As you see, load images from assets in Flutter is an easy task to handle and very controllable.
Full demo source code for this tutorial is put on Github, https://github.com/petehouston/flutter-image-asset
Check it out if you want to review the code.